Copy Link
Add to Bookmark
Report
Java Coffee Break Newsletter Volume 2 Issue 05
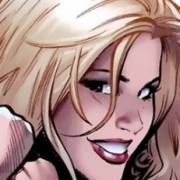
Java Coffee Break Newsletter Volume 2, Issue 5
http://www.davidreilly.com/jcb/
ISSN 1442-3790
=============================================================================
In this issue
* Java Book Selections
* Java in the news
* Q&A : How can I use a quote " character within a string?
* Article : Top Ten Errors Java Programmers Make
=============================================================================
Java Book Selections
Java Swing
by Robert Eckstein, Marc Loy & David Wood
With the introduction of the Swing graphical toolkit to Java 1.2, developers
now have the freedom to write applications with rich graphical user
interfaces (GUI). Swing gives Java applications the professional edge that
has long been shared by their C++, VB & Delphi counterparts, and goes further
with a huge range of new components and controls, and customizable
"look-and-feels". But while Swing may be the way of the future for
developers, its a steep learning curve because of the complexity of the
Swing toolkit. That's where "Java Swing", published by O'Reilly, comes in.
Java Swing, at a whopping 1200+ pages, is a fantastic reference that you'll
keep within arms reach as you program in Swing. But the book is more than
just an API reference - its a combined tutorial and book of examples. Aside
from the first few chapters, which provide a basic grounding for the rest of
the book, you can skip from chapter to chapter as your needs dictate. It
covers all the major component groups, as well as providing useful examples
and code snippets.
My one complaint about this title is that it appears to have tried covered
the entire swing library, and in doing so the authors tried to put just too
many topics into it, at the expense of detail. Perhaps it needs to be split
into two volumes, but in my programming I found that there were some areas
where a more thorough treatment should have been given (in particular, the
chapter on trees which provides not enough detail, and only very simple
examples). That said, "Java Swing" is a fantastic resource, both as a
reference and an overview/tutorial of Swing, and is one best Swing
references available.
[more] http://www.davidreilly.com/goto.cgi?isbn=156592455X
Pure Jfc : The Swing Toolkit (The Pure Series)
by Dr Satyaraj Pantham
In the past few months, I've read many books about Swing, the new graphics
library from Sun. One thing stands out above all others - a lack of good
code examples. Its not just that you can learn so much from looking at
someone else's code, or that code helps reinforce the theory taught by an
author. Good code examples, the type that are packed throughout
"Pure JFC Swing" can be used AFTER you've learnt the basic theory of Swing
- in your own applications, and projects.
That's the main reason why I'm so impressed with "Pure JFC Swing". Its
certainly a good reference for those wanting to learn more about Swing -
but there are many good references on the topic, such as "Java Swing".
It has a good API reference as well, but most books on Swing include a
lengthy API reference. What makes this book stand out from the crowd is
its code. Not only are there code examples for all of the components in
Swing, but these code examples are also far superior to any I've seen before.
They've well commented, and show the signs of someone intimately familiar
with Swing's inner-workings. Even complex topics, like trees, are given
comprehensive coverage (something that titles like Java Swing lack
significantly). For example, Dr Pantham shows you step by step how to create
trees, and tree models, and then finally how to provide your own custom
rendering of tree nodes and leafs.
The author's code examples are easy to understand, with good commentary.
Code examples start simple, and then build on previous examples as you
progress throughout each chapter. If you have a particular interest in a
component, you can go straight to it and within minutes have working code -
a big plus for those who are working to deadlines, and can't afford to read
ever chapter in sequence.
My one complaint with this title is that the code isn't provided on CD, and
though the code can be downloaded from the publisher's site, its a little hard
to find. Once you've downloaded it though, you're well on your way to
programming in Swing. All of the examples can be easily incorporated into
your own applications, so it is a great timesaver, and an excellent way to
improve the quality of your applications. If you've been disappointed, like
myself, by the examples in other Swing references, and want good working code
that demonstrates the Swing component library, this book is for you!
[more] http://www.davidreilly.com/goto.cgi?isbn=0672314231
=============================================================================
In the News
Here are a selection of recent news items that may be of interest to
Java developers.
/*/ Sun releases HotSpot performance engine
The much anticipated "HotSpot" performance engine is now available
for donwload. Sun claims it offers significantly faster performance
than normal "Just-in-Time" (JIT) compilers.
For more information, and to download HotSpot, visit
http://java.sun.com/products/hotspot/
/*/ Borland JBuilder 3 supports Java 2 platform
Borland JBuilder 3 will support the Java 2 platform, including
support for servlets, CORBA, and Enterprise JavaBeans. In addition
JBuilder will include an Open-Tools API, allowing seemless
integration of third party tools.
For more information, visit the Borland JBuilder site, at
http://www.borland.com/jbuilder/
=============================================================================
Q&A : How can I use a quote " character within a string?
When we use literal strings in Java, we use the quote (") character to indicate
the beginning and ending of a string. For example, to declare a string called
myString, we could this :-
String myString = "this is a string";
But what if we wanted to include a quote (") character WITHIN the string. We
can use the \ character to indicate that we want to include a special
character, and that the next character should be treated differently. \"
indicates a quote character, not the termination of a string.
public static void main (String args[])
{
System.out.println ("If you need to 'quote' in Java");
System.out.println ("you can use single \' or double \" quote");
}
This allows us to include quote characters within a string. Its not something
you'll need to do often, but when you need it, this tip will come in handy!
=============================================================================
Top Ten Errors Java Programmers Make
(How to spot them. How to fix/prevent them.)
Whether you program regularly in Java, and know it like the back of
your hand, or whether you're new to the language or a casual
programmer, you'll make mistakes. It's natural, it's human, and guess
what? You'll more than likely make the same mistakes that others do,
over and over again. Here's my top ten list of errors that we all seem
to make at one time or another, how to spot them, and how to fix them.
10. Accessing non-static member variables from static methods (such as main)
Many programmers, particularly when first introduced to Java, have problems
with accessing member variables from their main method. The method signature
for main is marked static - meaning that we don't need to create an instance
of the class to invoke the main method. For example, a Java Virtual Machine
(JVM) could call the class MyApplication like this :-
MyApplication.main ( command_line_args );
This means, however, that there isn't an instance of MyApplication - it
doesn't have any member variables to access! Take for example the following
application, which will generate a compiler error message.
public class StaticDemo
{
public String my_member_variable = "somedata";
public static void main (String args[])
{
// Access a non-static member from static method
System.out.println ("This generates a compiler error" +
my_member_variable );
}
}
If you want to access its member variables from a non-static method (like
main), you must create an instance of the object. Here's a simple example of
how to correctly write code to access non-static member variables, by first
creating an instance of the object.
public class NonStaticDemo
{
public String my_member_variable = "somedata";
public static void main (String args[])
{
NonStaticDemo demo = new NonStaticDemo();
// Access member variable of demo
System.out.println ("This WON'T generate an error" +
demo.my_member_variable );
}
}
9. Mistyping the name of a method when overriding
Overriding allows programmers to replace a method's implementation with new
code. Overriding is a handy feature, and most OO programmers make heavy use
of it. If you use the AWT 1.1 event handling model, you'll often override
listener implementations to provide custom functionality. One easy trap to
fall into with overriding, is to mistype the method name. If you mistype the
name, you're no longer overriding a method - you're creating an entirely new
method, but with the same parameter and return type.
public class MyWindowListener extends WindowAdapter {
// This should be WindowClosed
public void WindowClose(WindowEvent e) {
// Exit when user closes window
System.exit(0);
}
});
Compilers won't pick up on this one, and the problem can be quite frustrating
to detect. In the past, I've looked at a method, believed that it was being
called, and taken ages to spot the problem. The symptom of this error will be
that your code isn't being called, or you think the method has skipped over
its code. The only way to ever be certain is to add a println statement, to
record a message in a log file, or to use good trace debugger (like Visual
J++ or Borland JBuilder) and step through line by line. If your method still
isn't being called, then it's likely you've mistyped the name.
8. Comparison assignment ( = rather than == )
This is an easy error to make. If you're used other languages before, such as
Pascal, you'll realize just how poor a choice this was by the language's
designers. In Pascal, for example, we use the := operator for assignment, and
leave = for comparison. This looks like a throwback to C/C++, from which Java
draws its roots.
Fortunately, even if you don't spot this one by looking at code on the
screen, your compiler will. Most commonly, it will report an error message
like this : "Can't convert xxx to boolean", where xxx is a Java type that
you're assigning instead of comparing.
7. Comparing two objects ( == instead of .equals)
When we use the == operator, we are actually comparing two object references,
to see if they point to the same object. We cannot compare, for example, two
strings for equality, using the == operator. We must instead use the .equals
method, which is a method inherited by all classes from java.lang.Object.
Here's the correct way to compare two strings.
String abc = "abc"; String def = "def";
// Bad way
if ( (abc + def) == "abcdef" )
{
......
}
// Good way
if ( (abc + def).equals("abcdef") )
{
.....
}
The full article is available for free from the Java Coffee Break
http://www.davidreilly.com/jcb/articles/toptenerrors.html
=============================================================================
The Java Coffee Break Newsletter is only sent out to email subscribers
who have requested it, and to readers of the comp.lang.java.programmer
and comp.lang.java.help newsgroups.
If you'd like to receive our newsletter, and get the latest Java
news, tips and articles from our site, then get your FREE subscription
from
http://www.davidreilly.com/jcb/newsletter/
If you are an email subscriber and no longer wish to receive the JCB
Newsletter, please unsubscribe using the WWW form located at
http://www.davidreilly.com/jcb/newsletter/unsubscribe.html