Copy Link
Add to Bookmark
Report
Java Coffee Break Newsletter Volume 3 Issue 08
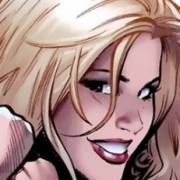
Java Coffee Break Newsletter Volume 3, Issue 8
http://www.javacoffeebreak.com/
ISSN 1442-3790
=================================================================
In this issue
* Featured Java Websites
* Book Review - Professional JSP
* Q&A : What is an abstract class, and when should it be used?
* Q&A : How do I kill a thread? The stop() method is deprecated
in JDK1.2, so how do I shut it down?
* Q&A : How can I append an existing file in Java?
=================================================================
\ /
\ JavaCon 2000 Enterprise Developers' Conference /
\ /
\ Experience four days of talks and presentations from /
/ leading industry experts on Java and Enterprise \
/ development, as well as an exciting exhibit hall with \
/ vendor presentations. For more information, email \
/ javacon2000@davidreilly.com September 24-27, 2000 \ \
Featured Java Websites
Here are a selection of websites that may be of interest to
readers.
ARGO - The Free UML Design Tool for Java
If you've put off using UML tools like Rational Rose because
of the expense, then have I got a great software package for
you! Argo is a FREE tool for UML design, for use with Java.
Coincidentally, it's also written in Java, so you can run it
on a Unix or a Windows box without any problems. The interface
uses Sun's Swing library, so it's very easy to use and highly
functional. There's even source code available for those who
like to tinker. Argo is a great tool, and you certainly can't
haggle about the price!
http://argouml.tigris.org/
Software Development Magazine Online
If you're a serious software developer, then you need
Software Development Magazine. Unlike many publications,
SDM puts their best articles online for free, and there's
an extensive archive of back-issues. There are articles on
programming techniques, debugging, tools and IDEs, software
design, and much more. There are also regular articles on
individual languages such as Java and C++. This is a great
read for developers, packed full of useful tips and tricks
to improve your software.
http://www.sdmagazine.com/
Get the lowdown on Java performance
Looking for some independent analysis on Java performance?
Performance varies dramatically from vendor to vendor -- so
which is the fastest?
Many benchmarking tests for Java are available of course,
but they often deliver conflicting results. The "Java
Performance Report," by Osvaldo Doederlein, offers an
objective analysis of all these benchmarks, so you can
make an informed decision. Want fast Java? This is the
report you have to read!
http://www.javalobby.org/features/jpr/
=================================================================
Book Review - Professional JSP
Author : Dan Malks, et al
Publisher : Wrox Press
ISBN : 1861003625
Experience: Beginner - Intermediate
For developers involved with web-based projects, whether it be an
online store for electronic commerce or an Intranet site for
accessing and modifying company data, the powerful blend of
JavaServer Pages (JSP) and Enterprise JavaBeans (EJB)
technologies can really make life simple. Once you've mastered
them, creating new components that encapsulate business logic,
or new web interfaces to existing systems, is easy. The trick,
for developers, is mastering the technologies.
Professional JSP is one way to get up to speed. Like many of the
books published by Wrox Press, Professional JSP covers a specific
technology in-depth, as well as the various ancillary topics
relating to it such as databases, servlets, and XML. While not
every developer will need every web technology covered by the
book (and there are many), the book works both as a tutorial to
cover the basics and a reference for technologies that you may
encounter later.
Professional JSP starts by covering the basics of Java Server
Pages, and how they relate to other web technologies. Embedded in
HTML pages, JSP provides an easy mechanism for creating
interactive web interfaces that draws on server-side components,
known as Enterprise JavaBeans. While the presentation logic is
written in JSP, the processing occurs within these JavaBean
components. The book takes a balanced approach, covering both
JSP and its syntax, as well as how to write and interact with
JavaBeans to perform useful tasks, like accessing databases
through JDBC and using other Java technologies. However, if
you've read other Wrox titles, you may find there is some overlap
in the topics covered.
One of the nice things about Professional JSP is that, in
addition to covering theory, it goes further and examines
practical applications of JSP, and issues for programmers like
security and debugging. Like other titles in the Professional
series, there are case studies of real projects using JSP and
related technologies. My favorite would have to be the case
study on porting Active Server Pages to JSP -- something that
is extremely important for developers with "legacy" web systems.
On the whole, Professional JSP is an excellent book for web
developers wanting to get up to speed with Java Server Pages,
web development, and Enterprise JavaBeans. However, developers
with less of a web presentation focus and more of back-end
server view may also want to consider the excellent
Professional Java Server Programming title, which also
covers JSP. -- David Reilly
For more information about this title, or to order it, visit
http://www.davidreilly.com/goto.cgi?isbn=1861003625
=================================================================
Q&A: What is an abstract class, and when should it be used?
Abstract classes are classes that contain one or more abstract
methods. An abstract method is a method that is declared, but
contains no implementation. Abstract classes may not be
instantiated, and require subclasses to provide implementations
for the abstract methods. Let's look at an example of an abstract
class, and an abstract method.
Suppose we were modeling the behavior of animals, by creating a
class hierachy that started with a base class called Animal.
Animals are capable of doing different things like flying,
digging and walking, but there are some common operations as
well like eating and sleeping. Some common operations are
performed by all animals, but in a different way as well.
When an operation is performed in a different way, it is a
good candidate for an abstract method (forcing subclasses to
provide a custom implementation). Let's look at a very primitive
Animal base class, which defines an abstract method for making
a sound (such as a dog barking, a cow mooing, or a pig oinking).
public abstract Animal
{
public void eat(Food food)
{
// do something with food....
}
public void sleep(int hours)
{
try
{
// 1000 milliseconds * 60 seconds *
// 60 minutes * hours
Thread.sleep ( 1000 * 60 * 60 * hours);
}
catch (InterruptedException ie) { /* ignore */ }
}
public abstract void makeNoise();
}
Note that the abstract keyword is used to denote both an
abstract method, and an abstract class. Now, any animal that
wants to be instantiated (like a dog or cow) must implement
the makeNoise method - otherwise it is impossible to create
an instance of that class. Let's look at a Dog and Cow subclass
that extends the Animal class.
public Dog extends Animal
{
public void makeNoise() {
System.out.println ("Bark! Bark!");
}
}
public Cow extends Animal
{
public void makeNoise() {
System.out.println ("Moo! Moo!");
}
}
Now you may be wondering why not declare an abstract class as an
interface, and have the Dog and Cow implement the interface. Sure
you could - but you'd also need to implement the eat and sleep
methods. By using abstract classes, you can inherit the
implementation of other (non-abstract) methods. You can't do
that with interfaces - an interface cannot provide any method
implementations.
=================================================================
Q&A: How do I kill a thread? The stop() method is
deprecated in JDK1.2, so how do I shut it down?
The API documentation for JDK1.2 discusses an alternate mechanism
for stopping threads, by having them continually poll a boolean
flag to see if they should terminate of their own accord. This is
an option, but if you have threads that become deadlocked or
stall waiting for I/O, sometimes you'll have to kill them the
hard way.
Why shouldn't you normally use the Thread.stop() method? Well, it
is deprecated as of JDK1.2 because it can potentially leave the
system in an unsafe state. If a thread had a lock on an object
(within a synchronized block), it might not release the object
lock, causing problems at a later time. Note that this problem
can affect older JVM implementations as well, JDK1.02 and JDK1.1
are not immune.
So while you shouldn't use Thread.stop() unless absolutely
necessary, it is an acceptable way to kill a thread if it
becomes stalled and you really need to shut it down fast.
=================================================================
Q&A : How can I append an existing file in Java?
Appending data to a file is a fairly common task. You'll be
surprised to know that there was no support for file appending
in JDK1.02, and developers supporting that platform are forced to
re-write the entire file to a temporary file, and then overwrite
the original. As most users support either JDK1.1 or the Java 2
platform, you'll probably be able to use the following
FileOutputStream constructor to append data:
public FileOutputStream(String name,
boolean append)
throws FileNotFoundException
Parameters: name - the system-dependent file name
append - if true, then bytes will be written to the
end of the file rather than the beginning
For example, to append the file 'autoexec.bat' to add a new path
statement on a Wintel machine, you could do the following:
FileOutputStream appendedFile = new FileOutputStream
("c:\\autoexec.bat", true);
=================================================================
The Java Coffee Break Newsletter is only sent out to email
subscribers who have requested it, and to readers of the
comp.lang.java.programmer and comp.lang.java.help
newsgroups.
If you'd like to receive our newsletter, and get the latest
Java news, tips and articles from our site, then get your FREE
subscription & back issues from
http://www.javacoffeebreak.com/newsletter/
If you are an email subscriber and no longer wish to receive
the JCB Newsletter, please unsubscribe by emailing
javacoffeebreak-unsubscribe@listbot.com